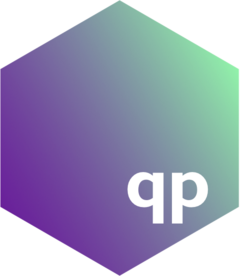
Add known concentrations of protein to standard samples
Source:R/qp_add_std_conc.R
qp_add_std_conc.Rd
Add known concentrations of protein to standard samples
Arguments
- x
A
data.frame
containing asample_type
andindex
columns. See details.- standard_scale
A numeric vector giving the concentrations of the standards. The units are arbitrary, but will determine the units of the output concentrations.
- ...
Unused
Details
Input is expected to have two columns:
sample_type
: A character vector denoting which samples are standards with "standard". All other values will be considered unknowns.index
: A numeric column denoting the sample number. Index 1 will correspond to the first item instandard_scale
, 2 will be the second, etc.
Examples
abs <- expand.grid(
sample_type = c("standard", "unknown"),
index = 1:7
)
abs
#> sample_type index
#> 1 standard 1
#> 2 unknown 1
#> 3 standard 2
#> 4 unknown 2
#> 5 standard 3
#> 6 unknown 3
#> 7 standard 4
#> 8 unknown 4
#> 9 standard 5
#> 10 unknown 5
#> 11 standard 6
#> 12 unknown 6
#> 13 standard 7
#> 14 unknown 7
qp_add_std_conc(abs)
#> sample_type index .conc
#> 1 standard 1 0.000
#> 3 standard 2 0.125
#> 5 standard 3 0.250
#> 7 standard 4 0.500
#> 9 standard 5 1.000
#> 11 standard 6 2.000
#> 13 standard 7 4.000
#> 2 unknown 1 NA
#> 4 unknown 2 NA
#> 6 unknown 3 NA
#> 8 unknown 4 NA
#> 10 unknown 5 NA
#> 12 unknown 6 NA
#> 14 unknown 7 NA
# Can add custom scale - doesn't have to be 'in order' or unique:
qp_add_std_conc(abs, c(1, 4, 2, 2, 3, 0.125, 7))
#> sample_type index .conc
#> 1 standard 1 1.000
#> 3 standard 2 4.000
#> 5 standard 3 2.000
#> 7 standard 4 2.000
#> 9 standard 5 3.000
#> 11 standard 6 0.125
#> 13 standard 7 7.000
#> 2 unknown 1 NA
#> 4 unknown 2 NA
#> 6 unknown 3 NA
#> 8 unknown 4 NA
#> 10 unknown 5 NA
#> 12 unknown 6 NA
#> 14 unknown 7 NA
# Will warn - more values in `standard_scale` than standard indices
# Will drop extra
qp_add_std_conc(abs, 1:8)
#> Warning: Not all standards in scale used
#> sample_type index .conc
#> 1 standard 1 1
#> 3 standard 2 2
#> 5 standard 3 3
#> 7 standard 4 4
#> 9 standard 5 5
#> 11 standard 6 6
#> 13 standard 7 7
#> 2 unknown 1 NA
#> 4 unknown 2 NA
#> 6 unknown 3 NA
#> 8 unknown 4 NA
#> 10 unknown 5 NA
#> 12 unknown 6 NA
#> 14 unknown 7 NA
# Will error - fewer values in `standard_scale` than standard indices
if (FALSE) {
qp_add_std_conc(abs, 1:6)
}